Why is the Flutter performance optimization so important?
A well-optimized app ensures smooth, responsive, and seamless user experiences, which are essential for keeping users engaged and satisfied. Optimizing Flutter app performance is essential because it directly enhances the user experience, ensuring that apps are fast, smooth, and responsive.
Flutter offers powerful tools and techniques to optimize app performance, ensuring a smooth and responsive user experience. Here are essential strategies to improve your Flutter app performance.
Best practices to optimize your Flutter app
1. Avoid rebuilding widgets and use StatelessWidgets
One way we can improve our Flutter app performance is to avoid rebuilding widgets when it’s unnecessary. Rebuilding widgets increases the load time. To minimize unnecessary rebuilds, you can use StatelessWidgets whenever possible, as they do not require the build method to be called frequently. Another effective technique is to make your widgets as small as possible. By breaking down your UI into smaller, more manageable widgets, you can isolate and control the parts of the interface that need to be updated. This practice, known as the "divide and conquer" approach, ensures that only the necessary widgets are rebuilt, reducing the workload on the rendering engine and improving performance.
2. Use asynchronous calls
Utilizing asynchronous calls in your Flutter app is crucial for maintaining a smooth and responsive user experience. You prevent the main UI thread from becoming blocked and unresponsive by offloading time-consuming operations such as network requests, database queries, or file I/O to asynchronous functions. This ensures that the app remains responsive to user interactions while background tasks are completed. Dart's async and await keywords make it easy to handle asynchronous operations. Additionally, employing FutureBuilder or StreamBuilder widgets allows you to efficiently manage and display data that is fetched asynchronously, further enhancing the app's performance and user experience.
3. Reduce unnecessary resources
Another way to optimize your Flutter app, it’s to avoid having unnecessary resources.
That can be achieved in several ways. One way is to dispose of the resources once they’re no longer used. You can achieve this by overriding the dispose method in your StatefulWidget and ensuring that any controllers, listeners, or other resources are properly cleaned up.
4. Periodically check your pubspec.yaml file
An effective strategy to reduce the number of resources is to check the packages that are being used. A good practice is to periodically check your pubspec.yaml file and remove any unused library or package.
Not only this would improve the performance but also the maintainability of the application.
5. Use a State Management Library
There are many state management libraries available to Flutter. Some of the most used are Bloc, Riverpod and Provider. Those libraries offer several benefits that can significantly improve the performance of your app. They provide efficient state management, clear separation of concerns between UI and business logic, scalability as your app grows, consistency in state management, and optimized resource usage. Integrating one of these libraries into your Flutter project can enhance the overall responsiveness and stability of your application.
6. Optimize the way images are loaded
Usually, an application requires image loading for some feature. One way in which this can be optimized is by using the CachedNetworkImage package. It allows the application to display images that are loaded from an URL, using cache memory to improve performance and reduce data usage.
7. Load your resources as they are needed
Lazy loading is a technique that involves delaying the loading of resources until they are actually needed, rather than loading them all at once during the initial app startup. This approach can significantly improve the performance of your Flutter app by reducing initial load times and minimizing memory usage. By loading resources like images, data sets, or heavy components on demand, you ensure that only the essential elements are loaded initially, which leads to a faster and smoother user experience.
The framework already offers some tools to achieve this. Lazy loading in Flutter can be effectively implemented using the Visibility and ScrollController classes, ensuring that resources are loaded only when they enter the viewport, thus optimizing performance and enhancing user experience.
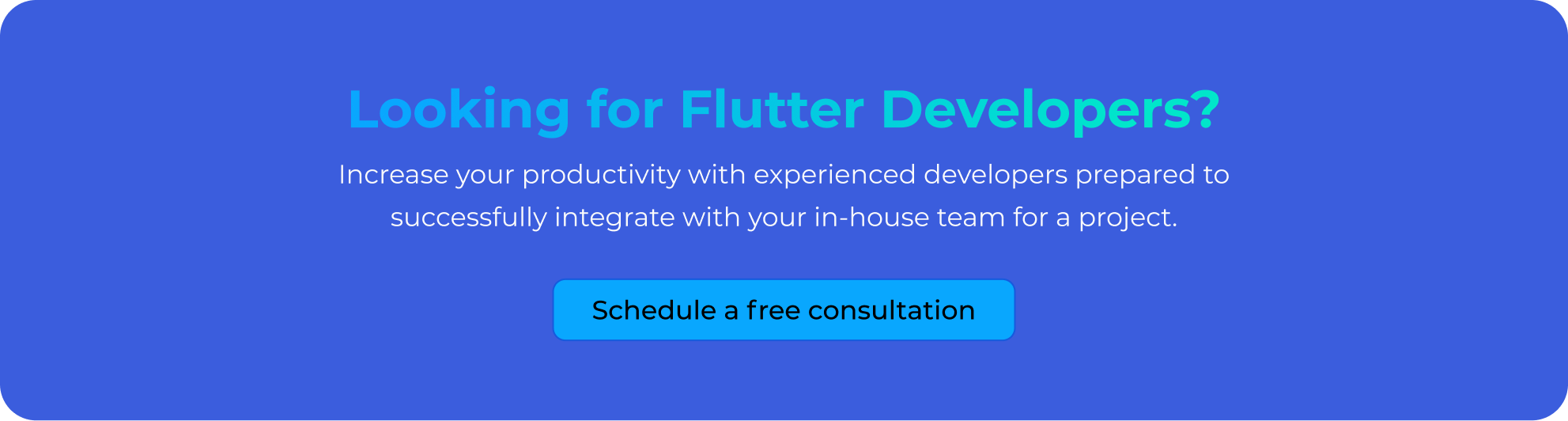
8. Avoid unnecessary uses of opacity and clipping
Some operations in Flutter are more expensive than others in terms of the resources they consume. Clipping and Opacity are one of those.
It is advised to use those operations only when it’s necessary. One way to avoid wrapping simple shapes or text in an Opacity widget is to just draw them with a semitransparent color. You can also implement fading to an image by using the FadeInImage widget, which applies a gradual opacity using the GPU's fragment shader.
Clipping is another expensive operation (not as expensive as opacity though). By default, clipping is disabled, so you must explicitly enable it when needed. To create a rectangle with rounded corners is recommended to use the borderRadius property offered by many of the widget classes.
9. Use Flutter Performance Tools
The framework already offers multiple performance optimization tools. For example, Flutter DevTools offers a visual and easy way of inspecting and analyzing an application. This tool offers the visualization of some metrics, such as CPU utilization, frame rate and memory usage. This can help developers to spot performance issues.
10. Use a code analyzer
Using code analyzers can provide developers with useful information on how their code could be improved. They help identify performance bottlenecks, enforce best practices, and ensure efficient state management. By using static and dynamic analysis, these tools can detect excessive widget rebuilds, memory leaks, and inefficient coding patterns. There are many analyzers like the “analyzer” package for Dart, which is a library that provides static analysis of the Dart code.
Another way in which the performance can be monitored is by using Codemagic. This is a powerful CI/CD tool that can significantly enhance the optimization process of Flutter apps. By integrating this into your Flutter project, you can automate the performance checks and ensure continuous monitoring of your app's performance metrics. This tool allows you to set up custom workflows that run automated tests, perform static code analysis, and generate performance reports.
11. Use the const keyword
The const keyboard is used to create compile-time constants. It can be used for widgets, strings, and even data models. Using it, we establish that the widget’s properties, the string or the data model involved will not be changed during their lifetime.
The use of this keyboard avoids unnecessary rendering so that the framework won’t check for changes,
12. Build method
The build method is called every time the framework needs to render the widget or when the configuration supplied to the build method changes. They can even be called when a parent widget is being rebuilt. That’s why It’s a good practice to avoid repetitive and costly work in build methods. Avoid calling for changes in higher parts of the widget tree and try to divide it into small widgets to avoid unnecessary rebuilds.
13. ListView.builder
The ListView.builder is useful when displaying large amounts of data.
This widget builder callback function is triggered selectively, processing only the items visible on the screen or soon to be visible, rather than generating all items simultaneously. This lazy loading approach optimizes performance by conserving resources until they are needed. Only visible elements occupy memory space.
Conclusion
Optimizing your Flutter app's performance isn't just about making it faster; it's about delivering a superior user experience. By using this Flutter performance best practices such as minimizing widget rebuilds, using asynchronous calls, and managing resources efficiently, developers can ensure their apps are responsive and resource-friendly. Integrating state management libraries, optimizing image loading, and implementing lazy loading techniques further enhance performance and scalability. Tools like Flutter DevTools and code analyzers provide invaluable insights for identifying and resolving performance bottlenecks early.
By prioritizing performance optimization throughout development and leveraging these strategies, developers can create high-quality Flutter applications that excel in responsiveness, reliability, and user satisfaction.